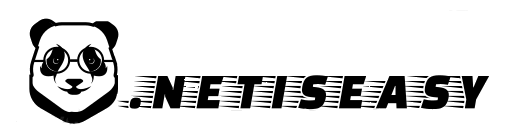
password validation in C#
Password validation in C#
public static bool ValidatePassword(string password, out string ErrorMessage)
{
bool reqUpperCaseLetter = true;
bool reqLowerCaseLetter = false;
bool reqDecimalDigit = false;
bool reqSpecialChar = false;
var input = password;
ErrorMessage = string.Empty;
if (string.IsNullOrWhiteSpace(input))
{
throw new Exception("Password should not be empty");
}
var hasNumber = new Regex(@"[0-9]+");
var hasUpperChar = new Regex(@"[A-Z]+");
var hasMiniMaxChars = new Regex(@".{8,15}");
var hasLowerChar = new Regex(@"[a-z]+");
var hasSymbols = new Regex(@"[!@#$%^&*()_+=\[{\]};:<>|./?,-]");
if (!hasLowerChar.IsMatch(input) && reqLowerCaseLetter ==true)
{
ErrorMessage = "Password should contain at least one lower case letter.";
return false;
}
else if (!hasUpperChar.IsMatch(input) && reqUpperCaseLetter ==true)
{
ErrorMessage = "Password should contain at least one upper case letter.";
return false;
}
else if (!hasMiniMaxChars.IsMatch(input))
{
ErrorMessage = "Password should not be lesser than 8 or greater than 15 characters.";
return false;
}
else if (!hasNumber.IsMatch(input) && reqDecimalDigit==false)
{
ErrorMessage = "Password should contain at least one numeric value.";
return false;
}
else if (!hasSymbols.IsMatch(input) && reqSpecialChar ==false)
{
ErrorMessage = "Password should contain at least one special case character.";
return false;
}
else
{
ErrorMessage = "Success";
return true;
}
}
Login for comment